React Native Skeleton Implementation
A barebones implementation of a skeleton can be created for react native using the existing Animated component and an external library for handling the gradient. In my implementation, I used expo-linear-gradient for this since my build uses expo. Below is the implementation.
Skeleton.tsx
Skeleton.tsx
import { useEffect, useRef } from 'react'; import { LinearGradient } from 'expo-linear-gradient'; import { Animated, View, StyleSheet, ViewStyle } from 'react-native'; type SkeletonProps = { width: number; height: number; style?: ViewStyle; }; export function Skeleton({ width, height, style, }: SkeletonProps) { const translateX = useRef(new Animated.Value(-width)).current; useEffect(() => { Animated.loop( Animated.timing(translateX, { toValue: width, useNativeDriver: true, duration: 1000, }) ).start(); }, [width]); return ( <View style={StyleSheet.flatten([ { width, height, backgroundColor: 'rgba(0,0,0,0.12)', overflow: 'hidden', }, style, ])} > <Animated.View style={{ width: '100%', height: '100%', transform: [{ translateX }] }} > <LinearGradient style={{ width: '100%', height: '100%' }} colors={['transparent', 'rgba(0, 0, 0, 0.05)', 'transparent']} start={{ x: 1, y: 1 }} /> </Animated.View> </View> ); }
This simple implementation is great for someone trying to replace their loading screens from using ActivityIndicator to a skeleton, without wanting to download several dependencies from an external library. The only limitation to this implementation is that a finite height and width must be set in order for the animation to work properly.
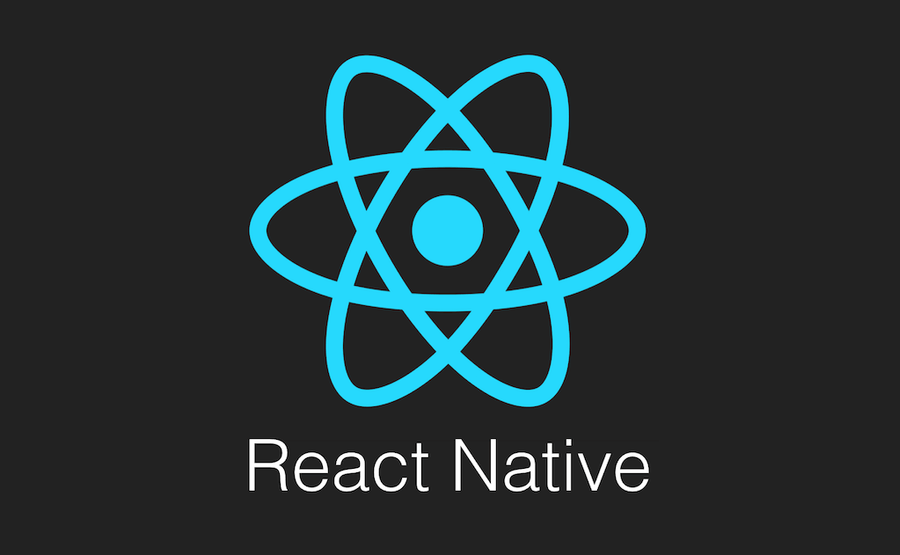
A barebones skeleton implementation compatible with expo on react native.
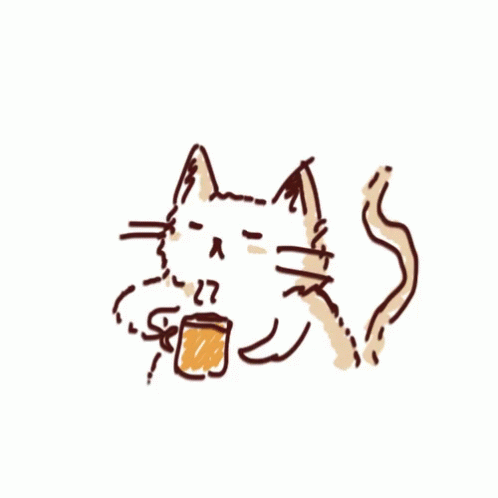
Jonathan Picazo
Avid Typer